Multi-Agent Runs in KaibanJS
Why Kanban for AI Agents?
Kanban is a tried-and-true methodology for managing work. We’ve adapted these concepts to meet the unique challenges of AI agent management.
If you've used tools like Trello, Jira, or ClickUp, you'll be familiar with how Kanban helps manage tasks.
Now, KaibanJS uses that same system to help you manage Multi-Agent AI systems in real-time.
The Kaiban Board: A Trello for AI Workflows
Transform console logs into visual, shareable workflows with the Kaiban Board. A familiar Trello-style playground that makes AI agents clear for your whole team and clients.
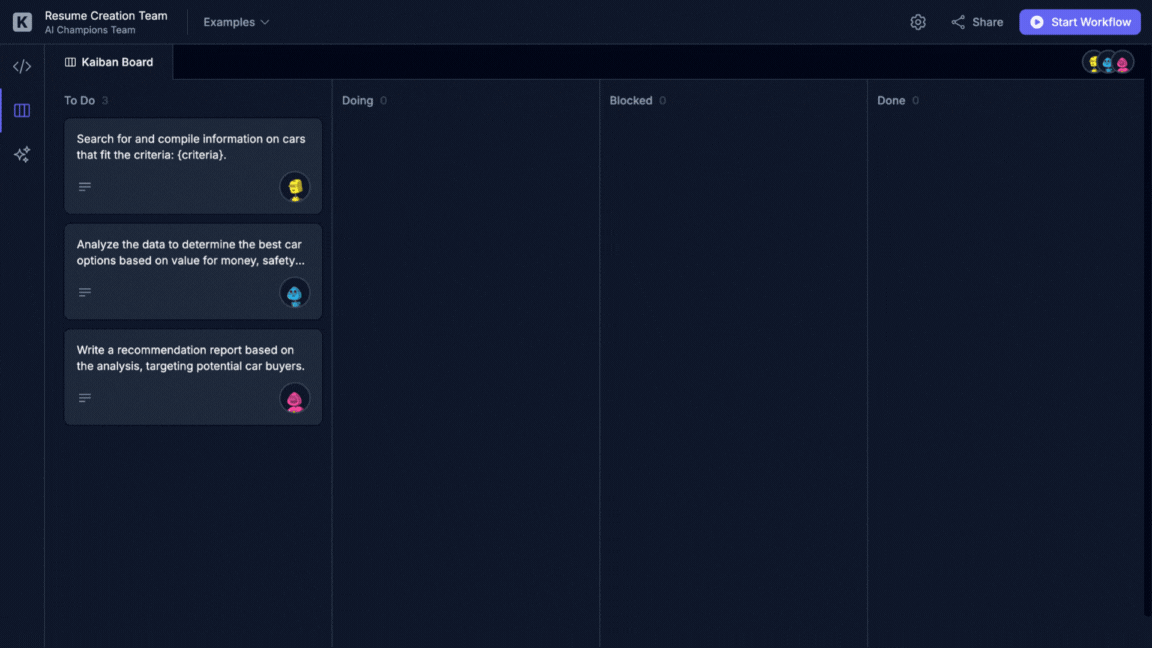
Run it locally, share with your team, and iterate quickly—without vendor lock-in.
Help us keep building!
Try it Yourself
Start the workflow to see AI agents in action on the Kaiban Board—just like Trello or Jira, but for AI agents and humans.
Like What You See?
Initial Setup
Initialize
Quickly set up
KaibanJS
.Terminalnpx kaibanjs@latest init
Run
Start the Kaiban Board locally.
Terminalnpm run kaiban
Or
For manual installation, follow the steps below.
Install
Install via npm or your preferred package manager.
Terminalnpm install kaibanjs
Import
Import
KaibanJS
in your JavaScript file using ES6 or CommonJS syntax.src/index.js// Using ES6 import syntax for NextJS, React, etc.
import { Agent, Task, Team } from "kaibanjs";
// Using CommonJS syntax for NodeJSconst { Agent, Task, Team } = require("kaibanjs");Create
Build your workflow using Agents, Tasks and a Team as building blocks.
src/index.js// Create agents, tasks, and the team
const developer = new Agent({goal: 'Implement UI features'
});const uiTask = new Task({
description: 'Develop the main interface',
});const devTeam = new Team({
agents: [developer],
tasks: [uiTask]
});devTeam.start();
Quick Start Video
Watch how to set up and run KaibanJS in less than a minute.
Get Started in Under 5 Minutes
Framework Quick Starts
React + AI Agents
Build and manage AI agents in your React applications with step-by-step guidance.
View DocsNode.js + AI Agents
Integrate AI agents into your Node.js backend with complete workflow examples.
View DocsNext.js + AI Agents
Create full-stack AI agent applications using Next.js and KaibanJS frameworks.
View DocsNew to AI Agents?
What Is an AI Agent?
An AI agent is like a specialized team member.
Just like a developer or designer in a team, an agent has a specific job — answering questions, processing data, or giving recommendations.
Key Features of KaibanJS
Integrate with Your Preferred JavaScript Frameworks
Easily add AI capabilities to your NextJS, React, Vue, Angular, and Node.js projects.
import React from 'react';import myAgentsTeam from './agenticTeam';
const TaskStatusComponent = () => {const useTeamStore = myAgentsTeam.useStore();
const { tasks } = useTeamStore(state => ({tasks: state.tasks.map(task => ({id: task.id,description: task.description,status: task.status}))}));
return (<div><h1>Task Statuses</h1><ul>{tasks.map(task => (<li key={task.id}>{task.description}: Status- {task.status}</li>))}</ul></div>);};
export default TaskStatusComponent;
For a deeper dive visit the documentation.
Your Support Fuels Our Progress
Robust State Management
KaibanJS employs a Redux-inspired architecture, enabling a unified approach to manage the states of AI agents, tasks, and overall flow within your applications. This method ensures consistent state management across complex agent interactions, providing enhanced clarity and control.
import myAgentsTeam from './agenticTeam';
const KaibanJSComponent = () => {const useTeamStore = myAgentsTeam.useStore();
const {agents, workflowResult } = useTeamStore(state => ({agents: state.agents,workflowResult: state.workflowResult,}));
return (<div><button onClick={myAgentsTeam.start}>Start Team Workflow</button><p>Workflow Result: {workflowResult}</p><div><h2>🕵️♂️ Agents</h2>{agents.map(agent => (<p key={agent.id}>{agent.name} - {agent.role} - Status: ({agent.status})</p>))}</div></div>);};
export default KaibanJSComponent;
For a deeper dive into state management with KaibanJS, visit the documentation.
Join The Journey
From Creation to Deployment: All in One Framework
KaibanJS covers every step of your AI journey-build, visualize, and easily integrate with your preferred deployment tools.
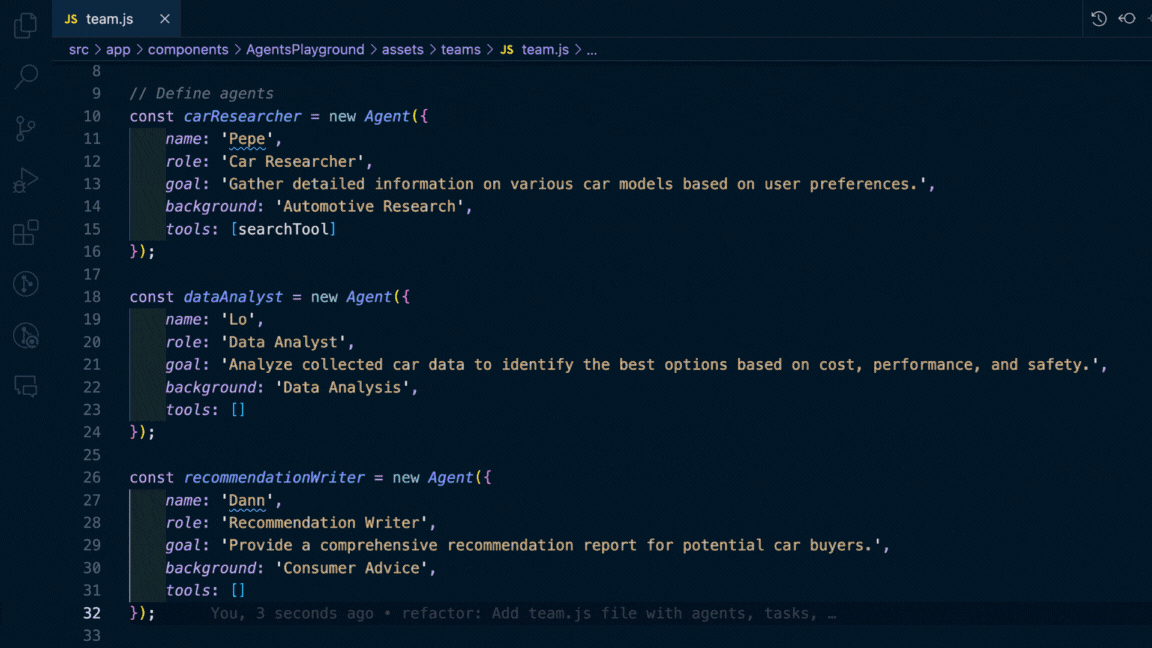
With KaibanJS, manage every step of the AI lifecycle. Create agents, visualize workflows, and integrate with deployment platforms like Vercel or AWS-all in one place.
We appreciate your support
Multiple LLMs Support
Optimize your AI solutions by integrating a range of specialized AI models, each tailored to excel in distinct aspects of your projects.
import { Agent } from 'kaibanjs';
const emma = new Agent({name: 'Emma',role: 'Initial Drafting',goal: 'Outline core functionalities',llmConfig: {provider: 'google',model: 'gemini-1.5-pro',}});
const lucas = new Agent({name: 'Lucas',role: 'Technical Specification',goal: 'Draft detailed technical specifications',llmConfig: {provider: 'anthropic',model: 'claude-3-5-sonnet-20240620',}});
const mia = new Agent({name: 'Mia',role: 'Final Review',goal: 'Ensure accuracy and completeness of the final document',llmConfig: {provider: 'openai',model: 'gpt-4o',}});
For further details on integrating diverse AI models with KaibanJS, please visit the documentation.
Help Us Grow Stronger
Your AI, Your Infrastructure
Run KaibanJS locally or deploy anywhere-no vendor lock—in, just full control.
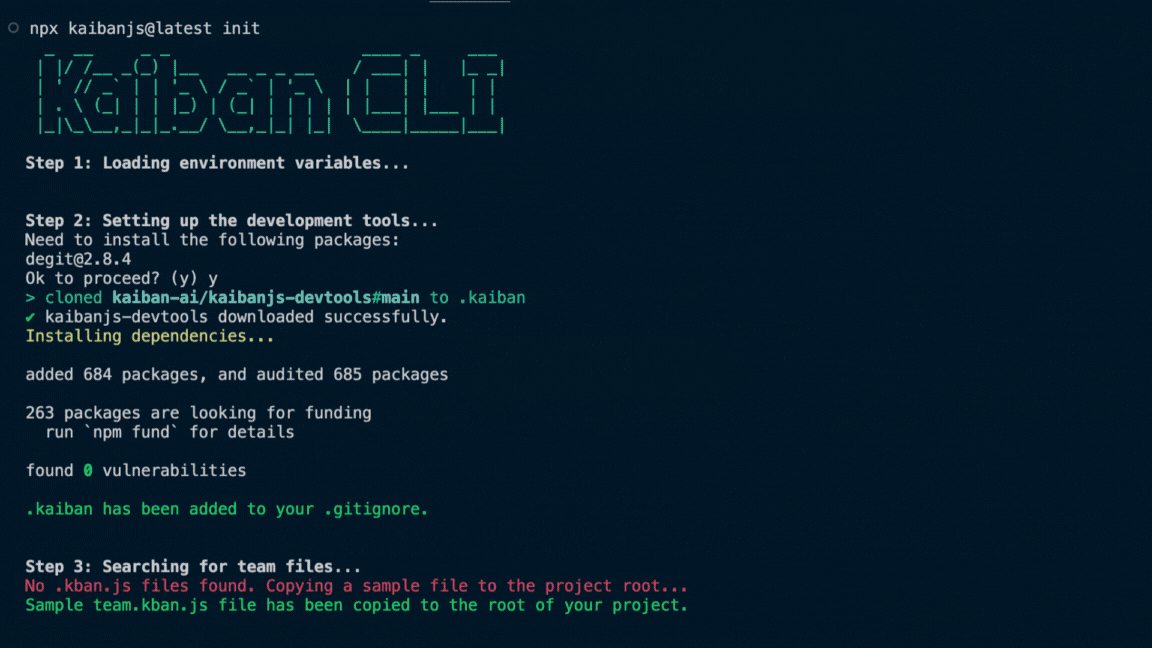
KaibanJS gives you complete ownership of your AI projects.
Run the Kaiban Board locally for fast iteration, or deploy it to your private servers-without relying on SaaS platforms.
Your support makes a difference
Role-Based Agent Design
Harness the power of specialization by configuring AI agents to excel in distinct, critical functions within your projects. This approach enhances the effectiveness and efficiency of each task, moving beyond the limitations of generic AI.
import { Agent } from 'kaibanjs';
const daveLoper = new Agent({name: 'Dave Loper',role: 'Developer',goal: 'Write and review code',background: 'Experienced in JavaScript, React, and Node.js',});
const ella = new Agent({name: 'Ella',role: 'Product Manager',goal: 'Define product vision and manage roadmap',background: 'Skilled in market analysis and product strategy',});
const quinn = new Agent({name: 'Quinn',role: 'QA Specialist',goal: 'Ensure quality and consistency',background: 'Expert in testing, automation, and bug tracking',});
For a deeper dive visit the documentation.
Your Star Means Everything
Tool Integration
Just as professionals use specific tools to excel in their tasks, enable your AI agents to utilize tools like search engines, calculators, and more to perform specialized tasks with greater precision and efficiency.
import { Agent, Tool } from 'kaibanjs';
const tavilySearchResults = new Tool({name: 'Tavily Search Results',maxResults: 1,apiKey: 'ENV_TRAVILY_API_KEY',});
const peterAtlas = new Agent({name: 'Peter Atlas',role: 'City Selector',goal: 'Choose the best city based on comprehensive travel data',background: 'Experienced in geographical data analysis and travel trends',tools: [tavilySearchResults],});
KaibanJS supports all LangchainJS-compatible tools, offering a versatile approach to tool integration. For further details, visit the documentation.
Your Star Makes a Difference
Observability and Monitoring
Built into KaibanJS, the observability features enable you to track every state change with detailed stats and logs, ensuring full transparency and control. This functionality provides real-time insights into token usage, operational costs, and state changes, enhancing system reliability and enabling informed decision-making through comprehensive data visibility.
const useStore = myAgentsTeam.useStore();
useStore.subscribe(state => state.workflowLogs, (newLogs, previousLogs) => {if (newLogs.length > previousLogs.length) {const { task, agent, metadata } = newLogs[newLogs.length - 1];if (newLogs} = newLogs[newLogs.length - 1].logType === 'TaskStatusUpdate') {switch (task.status) {case TASK_STATUS_enum.DONE:console.log('Task Completed',{taskDescription: task.description,agentName: agent.name,agentModel: agent.llmConfig.modelduration: metadata.duration,llmUsageStats: metadata.llmUsageStats,costDetails: metadata.costDetails,});break;case TASK_STATUS_enum.DOING:case TASK_STATUS_enum.BLOCKED:case TASK_STATUS_enum.REVISE:case TASK_STATUS_enum.TODO:console.log('Task Status Update',{taskDescription: task.description,taskStatus: task.status,agentName: agent.name});break;default:console.warn('Encountered an unexpected task status:', task.status);break;}}}});
For more details on how to utilize observability features in KaibanJS, please visit the documentation.
Together, We Build the Future
Real-Time Agentic Kanban Board
Work, prototype, run, and share your AI agents effortlessly with your teams and clients—no installations, complex commands, or servers required. Who said that AI is hard anymore?
Why a Kanban Board?
Kanban boards are excellent tools for showcasing team workflows in real time, providing a clear and interactive snapshot of each member's progress.
We've adapted this concept for AI agents.
Now, you can visualize the workflow of your AI agents as team members, with tasks moving from "To Do" to "Done" right before your eyes. This visual representation simplifies understanding and managing complex AI operations, making it accessible to anyone, anywhere.
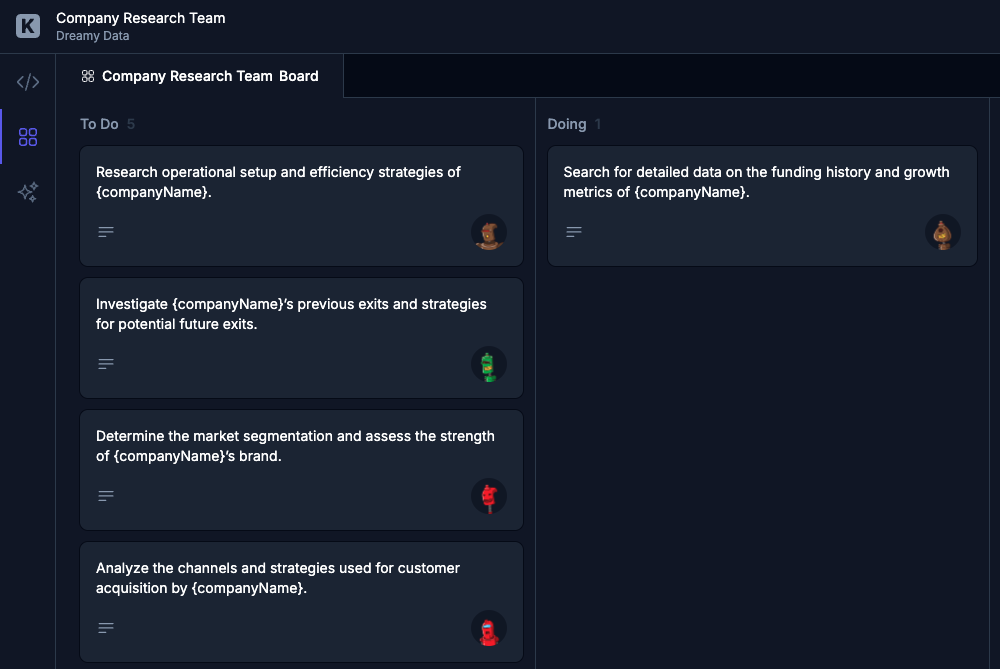
The Power of Agent-Based Workflows
By Andrew Ng
Today, we mostly use LLMs in zero-shot mode, prompting a model to generate final output token by token without revising its work.
This is akin to asking someone to compose an essay from start to finish, typing straight through with no backspacing allowed, and expecting a high-quality result.
Despite the difficulty, LLMs do amazingly well at this task!
With an agent workflow, however, we can ask the LLM to iterate over a document many times.
For example, it might take a sequence of steps such as:
- Plan an outline.
- Decide what, if any, web searches are needed to gather more information.
- Write a first draft.
- Read over the first draft to spot unjustified arguments or extraneous information.
- Revise the draft taking into account any weaknesses spotted.
- And so on.
This iterative process is critical for most human writers to write good text. With AI, such an iterative workflow yields much better results than writing in a single pass.
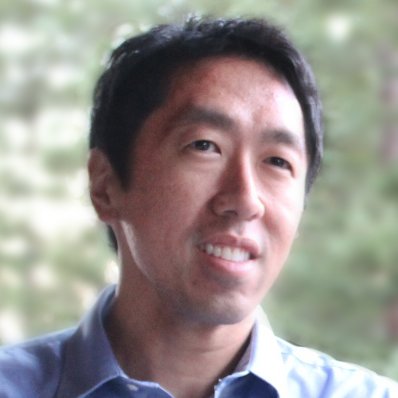
Developer Advantages of KaibanJS
Native JavaScript Development
Harness your existing JavaScript skills to manage and implement AI agents efficiently. KaibanJS fits right into your current workflow, minimizing the need to learn new technologies.
Human-Friendly API Design
KaibanJS is designed with simplicity at its core. It minimizes unnecessary complexity, making it easier for JavaScript developers to adopt and implement AI Agents into their projects.
Browser and Server Compatibility
KaibanJS operates seamlessly in any modern browser, leveraging client-side JavaScript effectively. It also extends to server-side environments with Node.js, enabling robust full-stack JavaScript development.
Compatible with React, Vue, Angular, NextJS and beyond...
KaibanJS seamlessly integrates with major front-end frameworks including React, Vue, Angular, and NextJS. This compatibility enhances functionality and ensures flexibility across various web development projects.
Production Ready
KaibanJS has been rigorously benchmarked for performance to ensure reliability and efficiency in production environments. Everyone is tired of cool-looking AI demos that don't deliver.
Enhanced Testing and Debugging
Thanks to its Redux-like state management architecture, KaibanJS offers robust tools and integrations that support comprehensive testing and effective debugging.
Developer Community
Join developers from world-class companies who trust KaibanJS
Meet Our Community
Developers from these companies have starred our GitHub repository
JavaScript Veterans on KaibanJS
Hear from experienced JavaScript veterans as they share their thoughts on the potential of KaibanJS to improve AI integration.
Reinier Guerra
Ex-Google, Ex-Salesforce
At Voxity.ai, we've leveraged AI to transform customer interactions fundamentally. Seeing the potential of KaibanJS, I am excited about how it could similarly revolutionize application development by making AI more accessible to JavaScript developers.
Its seamless integration and intuitive design could dramatically enhance how developers build interactive, responsive applications.
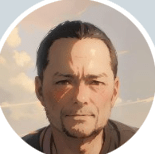
Arthur Sieler
Chief Developer at Cogency IO
Discovering KaibanJS has opened up exciting possibilities for Cogency IO. Its client-side capabilities fit perfectly into our system, allowing us to consider integrating advanced features directly into our Kanban boards without altering our Java backend.
I see tremendous potential in KaibanJS to streamline our operations and enhance productivity without complicating our existing infrastructure.
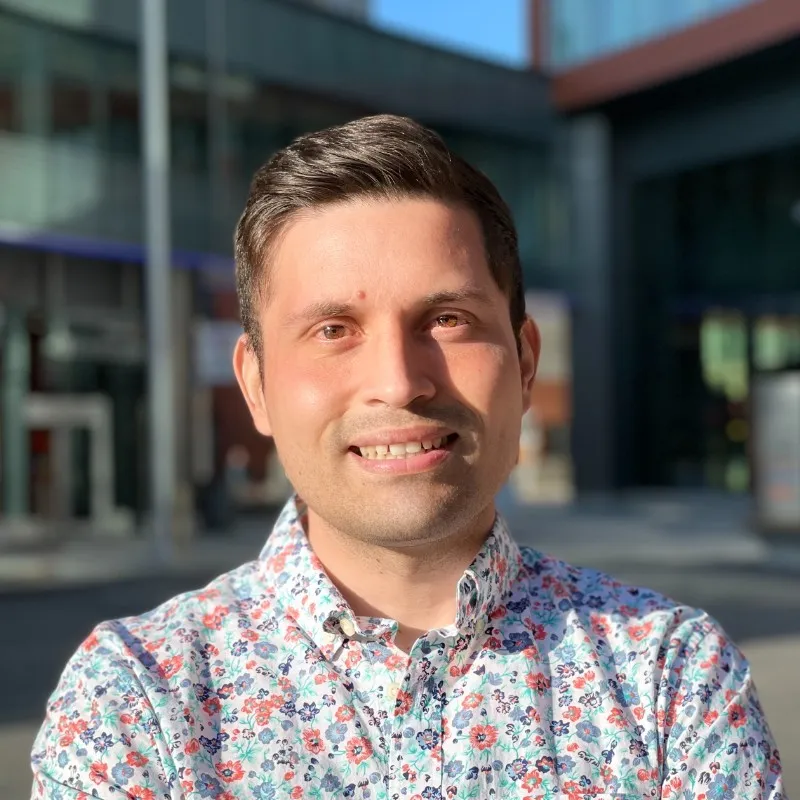
Jose Sandoya
Frontend Developer at Shopify
KaibanJS is a game-changer for developers who want to leverage AI without stepping out of their JavaScript ecosystem.
While I appreciate Python for its AI capabilities, it's incredibly convenient to stay within JavaScript when integrating AI agents into existing projects.
About Us
Hi there 👋, we are the KaibanJS team.
- We didn't graduate from @Harvard, @MIT, or any Ivy League school.
- We haven't worked at @Google, @Meta, @Amazon or any of the biggest tech companies.
- Nor have we been part of any big accelerator or VCs like @ycombinator, @sequoia, etc.
So our chances to push this framework forward are smaller compared with other teams.
(FIXME: Yep, believe it or not, there is some sort of elitism in the Startup scene.)
But...
We love what we do, have years of experience building complex web systems, and are ready to work hard.
Acknowledgments
We are deeply grateful to Andrew Ng and Andrej Karpathy, who inspire us as role models through their dedication to the AI community.
Thanks to João Moura, CrewAI, and the LangChain Team for their innovative ideas that inspire our work; to the Built in Miami team for their essential support through our accelerator program; to OpenAI and Anthropic for pioneering the technologies fundamental to our development.
Special thanks also to Anton and Seth for their invaluable advice and mentorship, and to all the friends, family, and members of the KaibanJS community who continually back and support us.
We’re almost there! 🌟 Help us hit 100 stars!
Star KaibanJS - Only 100 to go! ⭐